What is needed
- Setup an Azure AAD Application
- Add the web URIs which are allowed to use the OAuth2 login
- Add a client secret for your application
- Add API Permission to read the profile
- Add needed Azure dependencies
- Setup Spring OAuth config
- Adjust the CORS settings in Spring to ensure token refreshes
- Configure spring security
Setup an Azure AAD Application
First we need an Azure AAD application which gives us the access to our AD users. The important part are the client id and the tenant id here:
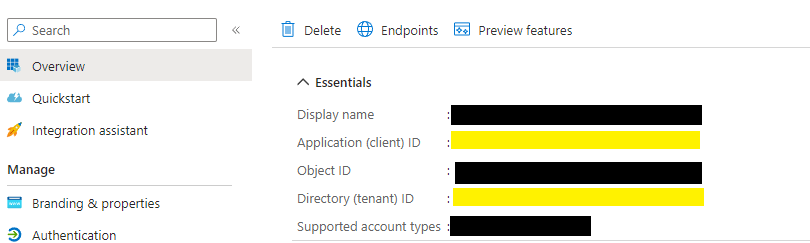
Add the web URIs which are allowed to use the OAuth2 login
In the next step we have to allow „applications“ based on their „DNS“ name to access and login through this AAD. Ensure to remember the yellow part, which is later needed in the configutation.
The URLs are basically the one you whitelist for a login. If you setup the „test“ AAD application you may want also add sometimes the localhost for testing. Ensure to remove it again later on.
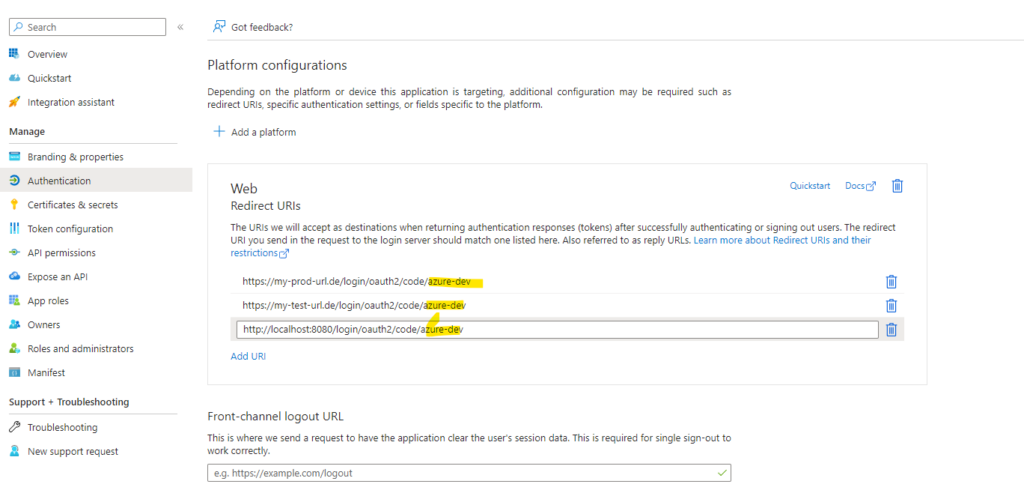
Add a client secret for your application
Next we have to create a secret for our application, we only require later the value of it. Please make sure you provide a new one after the expiration period.
You can have multiple secrets for multiple apps here.
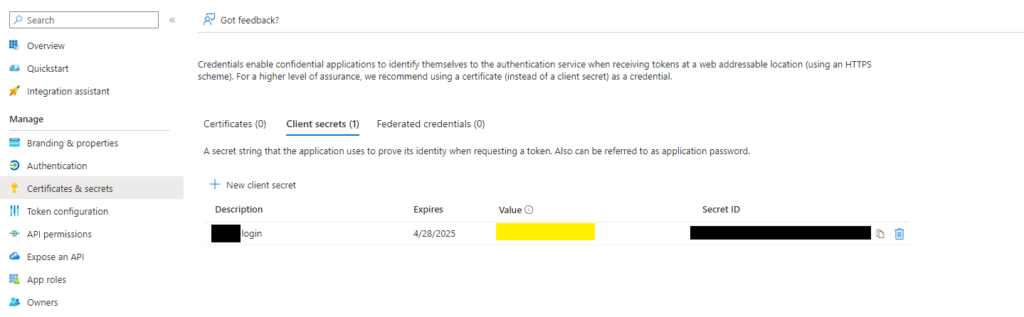
Setup Spring OAuth config
In this configuration we have to enter all the collected data azure-dev as name is here the suffix provided in the authentication section of the Azure AAD application. You can choose what ever name you like.
spring: security: oauth2: client: provider: azure: issuer-uri: https://login.microsoftonline.com/<Directory (tenant) ID>/v2.0 user-name-attribute: name registration: azure-dev: provider: azure client-id: <Application (client) ID> client-secret: <the secret value you created for your app> client-authentication-method: client_secret_post scope: - openid - email - profile
Spring Security
@Bean SecurityFilterChain filterChain(HttpSecurity http, OptionalYourUserService userService) throws Exception { // @formatter:off http .authorizeHttpRequests(authorizeHttpRequests -> authorizeHttpRequests // public URLs .requestMatchers(antMatcher("/actuator/health/readiness")).permitAll() // secure actuator .requestMatchers(antMatcher("/actuator/**")).hasRole(UserGroup.ADMIN) // app urls .requestMatchers("/api/**").authenticated() // default; recommended to use .authenticated() .anyRequest().permitAll() ).headers(headers -> headers.frameOptions(FrameOptionsConfig::sameOrigin)) .oauth2Login( oauth2 -> oauth2 .userInfoEndpoint(userInfo -> userInfo .oidcUserService(oidcUserService(userService))) ) .headers(headers -> headers.frameOptions(FrameOptionsConfig::sameOrigin)); // @formatter:on return http.build(); } private OAuth2UserService<OidcUserRequest, OidcUser> oidcUserService( OptionalYourUserService userService) { return userRequest -> { var oidcUser = new OidcUserService().loadUser(userRequest); // Delegate to the default // implementation // add any custom user service integration here, if required. return oidcUser; }; }
Add CORS Config
To avoid any CORS error
/ strict-origin-when-cross-origin
in your application we have to whitelist the OAuth2 provider for any redirect, in this example login.microsoft:
@Bean protected CorsConfigurationSource corsConfigurationSource() { CorsConfiguration configuration = new CorsConfiguration(); configuration.setAllowedOrigins(Arrays.asList("https://login.microsoftonline.com/**")); configuration.addAllowedHeader("*"); configuration.addAllowedMethod("*"); configuration.setAllowCredentials(true); UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource(); source.registerCorsConfiguration("/**", configuration); return source; }
Links
- https://learn.microsoft.com/en-us/azure/developer/java/spring-framework/spring-boot-starter-for-azure-active-directory-developer-guide